Quick Summary: Dive into React Flux with this comprehensive guide. Understand the core principles, architecture, and implementation of Flux in React applications. Learn how to manage application state effectively and build more maintainable, scalable applications.

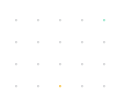
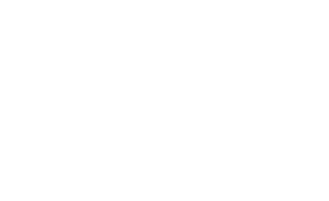
Hire top-notch React developers
Take your web development to the next level with our talented team of ReactJS developers
Introduction
React is a JavaScript library for building user interfaces, primarily focusing on building components. It’s maintained by Facebook and a community of individual developers and companies. React can be used as a base in the development of single-page or mobile applications. However, React concerns itself only with rendering data to the DOM, and so creating React applications usually requires the use of additional libraries for state management, routing, and other functionalities.
Flux is an application architecture that Facebook uses internally to build client-side web applications. It complements React's composable view components by utilizing a unidirectional data flow. Unlike MVC or two-way data binding systems, Flux is more rigid and emphasizes a clear separation of concerns.
Key Concepts of Flux
Actions
- Actions are simple objects that contain information about an event that has occurred (e.g., user interactions, network responses, system events).
- Actions have a type property and additional data needed to handle that event.
Dispatcher
- The dispatcher is a central hub that manages all data flow in a Flux application.
- It receives actions and dispatches them to the stores.
- Acts as a registry of callbacks that the stores can register with to receive actions.
Stores
- Stores hold the application state and logic.
- They register with the dispatcher and provide callbacks to handle actions.
- Stores update themselves based on the action's data and emit change events to notify the views of updates.
Views
- Views are React components that listen for changes from the stores.
- When a store updates, it emits a change event that the view listens for, causing the view to re-render with the new data.
Advantages of Using Flux with React
Predictable State Management
- Unidirectional data flow makes it easier to understand how data changes in the application.
- State changes are centralized in stores, making debugging and maintenance easier.
Decoupled Components
- Views and stores are decoupled; stores contain application logic and state, while views focus on rendering.
- This separation of concerns leads to more modular and reusable code.
Scalability
- Flux scales well in large applications because it enforces a clear structure.
- Centralized dispatching of actions allows for consistent state management.
Example: Simple Flux Application
Here’s a simple example of a Flux application managing a list of items:
- Action:
- Dispatcher:
- Store:
- View:
Advantages of React Flux
Predictable State Management:
- Unidirectional Data Flow: Flux's unidirectional data flow ensures that the state changes are predictable. Data flows in a single direction: from actions to the dispatcher, then to the stores, and finally to the views.
- Easier Debugging: Since the flow of data is straightforward and predictable, it is easier to track down bugs and understand where and why state changes occurred.
Decoupled Components:
- Separation of Concerns: Flux promotes the separation of concerns by decoupling the state management from the view logic. Stores manage the state and logic, while views render the data.
- Reusability: This separation makes components more reusable and easier to test, as they are not tightly coupled with the application’s state management.
Scalability:
- Structured Architecture: Flux provides a clear and structured way to manage application state, making it easier to scale large applications.
- Centralized Dispatching: With a centralized dispatcher handling all actions, the application’s state management becomes more organized, which is particularly beneficial in large-scale applications.
Consistency:
- Single Source of Truth: Stores act as the single source of truth for the application’s state. This centralization leads to consistency in state management across the application.
Improved Maintainability:
- Modular Code: Flux encourages writing modular code, which improves maintainability. Individual components and stores can be updated, extended, or replaced without affecting the entire application.
Disadvantages of React Flux
Complexity:
- Boilerplate Code: Implementing Flux requires a significant amount of boilerplate code, including setting up actions, the dispatcher, and stores. This can make the initial setup more complex compared to other state management solutions.
- Learning Curve: For developers new to Flux, there is a steep learning curve. Understanding the flow of data and the interactions between actions, dispatchers, and stores requires time and practice.
Verbosity:
- Redundancy: The structure of Flux can lead to verbose code with a lot of repetitive patterns. Every action needs to be dispatched, registered, and handled, which can result in a lot of boilerplate code.
Lack of Flexibility:
- Rigid Structure: While the unidirectional data flow provides predictability, it also imposes a rigid structure. This can be limiting in certain scenarios where more flexibility in state management might be beneficial.
Performance Considerations:
- Frequent Re-rendering: Since views re-render when stores emit change events, there could be performance issues in cases where the state changes frequently. Optimizing re-renders and managing performance can become complex in large applications.
Fragmented Ecosystem:
- Multiple Implementations: Flux is not a library but an architectural pattern, leading to multiple implementations (e.g., Facebook's Flux, Redux, Reflux). This can cause fragmentation and confusion about which implementation to choose.
Conclusion
React Flux offers a structured and predictable way to manage the application state, which is especially beneficial for large and complex applications. Its unidirectional data flow and separation of concerns enhance maintainability and scalability. However, the verbosity, complexity, and rigid structure can be challenging, particularly for smaller projects or teams new to the pattern. Understanding these trade-offs is crucial when deciding whether to implement Flux in a React application.
Looking for the best talent to bring your web projects to life? Enhance your digital journey with our Reactjs development company. Don't miss out on the opportunity to work with the top professionals in the field. Let's build something amazing together!