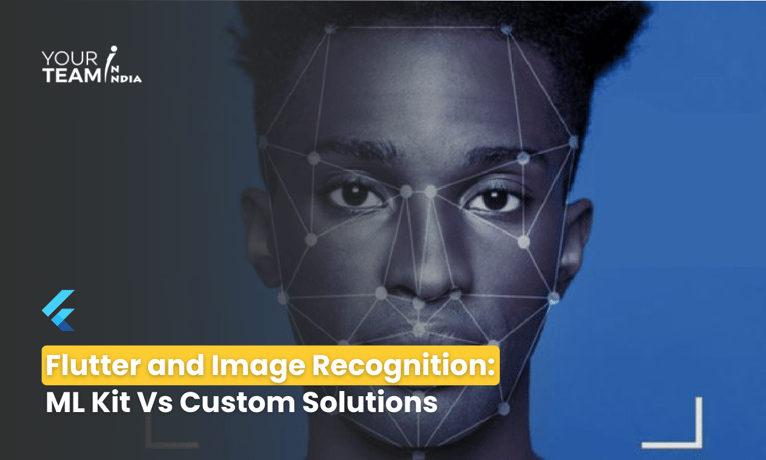
Quick Summary: Explore the world of image recognition in Flutter with this comprehensive guide comparing ML Kit and custom solutions. Learn the strengths, use cases, and implementation techniques of both approaches to enhance your Flutter apps with powerful image recognition capabilities.
Introduction
- The significance of image recognition in mobile applications.
- Overview of available solutions for image recognition in Flutter.
- Introduction to Google's ML Kit and the consideration of custom solutions.
Understanding ML Kit for Image Recognition
- Overview of ML Kit
- Recap of ML Kit as Google's machine learning SDK for mobile apps.
- Introduction to ML Kit's image recognition capabilities.
- Supported Features in ML Kit
- List of image recognition features provided by ML Kit.
- Examples of use cases, such as image labeling, text recognition, face detection, and more.
ML Kit: Pros and Cons
- Pros of ML Kit for Image Recognition
- Ease of integration with Flutter apps.
- Pre-trained models for various image recognition tasks.
- Support for on-device and cloud-based image processing.
- Continuous improvements and updates by Google.
- Cons of ML Kit for Image Recognition
- Limited customization compared to custom solutions.
- Dependency on network connectivity for cloud-based processing.
- May not cover highly specialized or niche use cases.
Implementing Image Recognition with ML Kit in Flutter
- Adding ML Kit Dependency
- Adding the firebase_ml_vision plugin to the Flutter project.
- Configuring the pubspec.yaml file.
# pubspec.yaml |
// Importing ML Kit package |
Using ML Kit for Image Labeling
- Implementing image labeling with ML Kit in Flutter.
- Extracting labels from images.
// Example: Image labeling with ML Kit |
Custom Solutions for Image Recognition
- Why Consider Custom Solutions?
- Flexibility and customization opportunities.
- Tailoring image recognition models to specific requirements.
- Handling highly specialized use cases.
- Creating Custom Image Recognition Models
- Overview of creating custom image recognition models.
- Using popular frameworks like TensorFlow or PyTorch.
// Example: Using a custom image recognition model |
Custom Solutions: Pros and Cons
- Pros of Custom Solutions for Image Recognition
- High level of customization and control over the model.
- Ability to handle specialized use cases and domain-specific requirements.
- Possibility to train and fine-tune models for improved accuracy.
- Cons of Custom Solutions for Image Recognition
- Steeper learning curve for model creation and training.
- Resource-intensive process for model development and maintenance.
- Requires domain expertise in machine learning.
Choosing Between ML Kit and Custom Solutions
- Decision Criteria
- Use case and application requirements.
- Level of customization needed.
- Development resources and expertise available.
- Consideration of real-time processing, offline capabilities, and resource constraints.
- Scenarios for ML Kit
- Rapid development with pre-trained models.
- General image recognition tasks.
- Projects with limited resources and time constraints.
- Scenarios for Custom Solutions
- Highly specialized or domain-specific requirements.
- Advanced customization and control over the model.
- Availability of machine learning expertise.
Let's create a simple example that compares image labeling using ML Kit and a custom solution in Flutter. This example will focus on labeling objects in an image.
ML Kit Image Labeling Example:
import 'package:flutter/material.dart'; |
Custom Image Labeling Example (using TensorFlow Lite):
This example assumes you have a custom TensorFlow Lite model for image labeling. You'll need to replace 'path/to/your/custom_model.tflite' with the path to your custom model file.
import 'package:flutter/material.dart'; |
In these examples, _labelImageWithMLKit and _labelImageWithCustomModel are functions triggered by buttons. These functions process an image and print the labels with confidence scores. The ML Kit example uses the Firebase ML Vision package, and the custom solution example uses the Tflite package for TensorFlow Lite integration. Adjust the paths and model loading accordingly based on your setup.
Conclusion
- Recap of the pros and cons of using ML Kit vs. custom solutions for image recognition in Flutter.
- Guidance on choosing the right approach based on specific project requirements.
- Encouragement for developers to explore and leverage the strengths of both ML Kit and custom solutions in their Flutter applications.
Hire Flutter developers to elevate your Flutter app design. Unlock the full potential of Flutter layouts with our professional Flutter developers.