Quick Summary: Master destructuring in ReactJS with this comprehensive guide. Learn how to simplify your code, improve readability, and manage state and props more effectively using destructuring techniques in React.

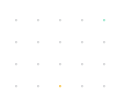
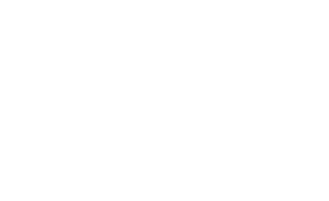
Hire top-notch React developers
Take your web development to the next level with our talented team of ReactJS developers
Introduction
Destructuring is a powerful feature in JavaScript that allows you to extract values from arrays and objects into distinct variables. This feature is particularly useful in ReactJS, where it can greatly enhance the readability and efficiency of your code. In this guide, we will explore how to effectively use destructuring in ReactJS, starting from the basics and advancing to more complex applications.
What is Destructuring?
Destructuring is a syntax introduced in ES6 that simplifies the process of extracting values from arrays and objects. Instead of accessing each property individually, destructuring allows you to unpack values directly from the source.
Example: Array Destructuring
Example: Object Destructuring
Destructuring in React Components
Destructuring is particularly useful in React components, where props and state are commonly used. It allows for cleaner and more readable code, reducing the need for repetitive references to props and state.
Destructuring Props
Instead of accessing props with this.props, you can destructure them directly in the function parameter or within the function body.
Destructuring State
When using the useState hook in functional components, destructuring allows you to separate state variables and their corresponding setters.
Advanced Destructuring Techniques
Destructuring can be used in various advanced scenarios to improve code clarity and efficiency.
Nested Destructuring
When dealing with nested objects, you can destructure multiple levels deep.
Destructuring with Default Values
You can set default values while destructuring to avoid undefined values.
Destructuring in Function Parameters
Destructuring can be directly applied in function parameters, making the function signature more concise.
Advantages
- Improved Readability
- Concise Syntax: Destructuring reduces the amount of code needed to access properties, making it more concise and easier to read.
- Clarity: By clearly defining the variables being used, it makes the code more transparent and understandable.
- Reduced Code Repetition
- Less Boilerplate: You don't need to repeatedly write props or state before every variable, which reduces boilerplate code.
- Convenience and Simplicity
- Direct Access: Directly access required values without the need for multiple lines of code.
- Default Values: Easily set default values for variables while destructuring, providing fallback values when data might be undefined.
- Enhanced Maintainability
- Consistent Structure: Encourages a consistent structure in how data is accessed, making it easier to manage and refactor code.
- Simplified Parameter Handling: Function parameters can be destructured directly, making function signatures simpler and more readable.
- Enhanced Performance
- Efficiency: Directly extracting required values can slightly improve the performance of the code as it avoids repetitive lookups.
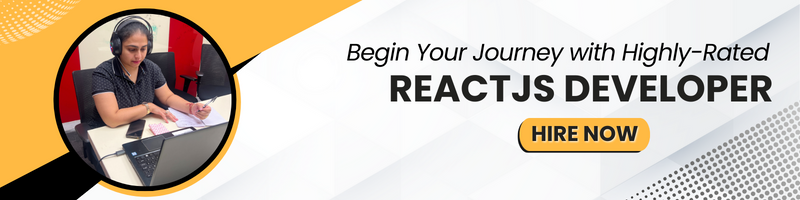
Disadvantages
- Potential for Overuse
- Excessive Destructuring: Overusing destructuring, especially in deeply nested objects, can lead to complicated and hard-to-read code.
- Confusion: New developers or those unfamiliar with destructuring might find the code harder to understand.
- Debugging Challenges
- Hidden Context: Destructuring hides the source of the data, which might make debugging more challenging if the origin of the values isn't clear.
- Silent Failures: If properties are renamed or removed, destructuring can fail silently, leading to bugs that are hard to trace.
- Naming Conflicts
- Variable Collisions: Destructured variables must have unique names, which can lead to naming conflicts and confusion in large codebases.
- Loss of Context: Losing the context of the original object can sometimes make the code less intuitive.
- Performance Overhead
- Shallow Copy: While destructuring generally improves performance, destructuring large objects can incur a performance cost due to the creation of shallow copies.
- Increased Complexity: For very simple components, destructuring might add unnecessary complexity.
- Learning Curve
- Initial Complexity: For beginners, understanding and effectively using destructuring can add to the learning curve, especially when combined with other advanced ES6 features.
Conclusion
Destructuring in ReactJS offers numerous advantages, such as improved readability, reduced code repetition, and enhanced maintainability. However, it also comes with potential drawbacks like overuse, debugging challenges, and a steeper learning curve for new developers. Understanding both the benefits and limitations of destructuring will help you apply it effectively and appropriately in your ReactJS projects, balancing code simplicity and clarity with the need for maintainability and performance.
Looking for the best talent to bring your web projects to life? Enhance your digital journey with our Reactjs development company. Don't miss out on the opportunity to work with the top professionals in the field. Let's build something amazing together!