Quick Summary: Integrating GeoJSON files in a Flutter app allows you to display markers on maps efficiently. This guide walks you through loading and rendering GeoJSON data to enhance map-based features in your Flutter applications, offering a seamless user experience with dynamic location data.

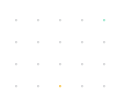
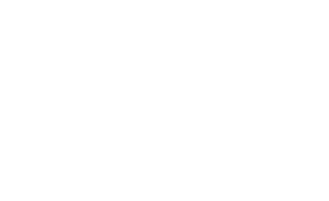
Ready to supercharge your app development with Flutter?
Hire Flutter Developers from YTII and embrace the future of app development.
Introduction
Flutter, Google’s UI toolkit for building natively compiled applications, has a rich set of libraries and plugins for integrating maps and displaying geospatial data. One common task is to load a GeoJSON file and display markers on a map at specific latitude and longitude coordinates. This guide will walk you through the process of achieving this using the flutter_map plugin and the ‘geoflutterfire’ package.
Prerequisites
Before we start, ensure you have the following:
- Flutter installed on your machine.
- Basic knowledge of Dart and Flutter.
- A GeoJSON file with the required data.
- Openstreet map key.
Step 1: Set Up Your Flutter Project
First, create a new Flutter project or open an existing one:
flutter create geojson_map cd geojson_map |
Open the pubspec.yaml file and add the necessary dependencies:
dependencies: flutter: sdk: flutter flutter_map: ^3.1.0 latlong2: ^0.8.1 http: ^0.13.4 geojson: ^0.3.0 |
Run flutter pub get to install the dependencies.
Step 2: Prepare Your GeoJSON File
For this tutorial, let's assume you have a GeoJSON file named data.geojson located in the assets directory of your project. Ensure you declare the assets directory in your pubspec.yaml:
flutter: assets: - assets/data.geojson |
Step 3: Load and Parse the GeoJSON File
Create a Dart file, e.g., geojson_loader.dart, to handle loading and parsing the GeoJSON file:
import 'dart:convert'; import 'package:flutter/services.dart' show rootBundle; import 'package:geojson/geojson.dart'; Future<List<GeoJsonPoint>> loadGeoJsonPoints() async { final String response = await rootBundle.loadString('assets/data.geojson'); final Map<String, dynamic> data = json.decode(response); GeoJson geoJson = GeoJson(); geoJson.processedPoints.listen((GeoJsonPoint point) { print('Processed point at ${point.geoPoint.latitude}, ${point.geoPoint.longitude}'); }); geoJson.endSignal.listen((_) => geoJson.dispose()); await geoJson.parse(data); return geoJson.points; } |
Step 4: Display Markers on the Map
Open the main.dart file and set up the map with markers:
import 'package:flutter/material.dart'; import 'package:flutter_map/flutter_map.dart'; import 'package:latlong2/latlong.dart'; import 'geojson_loader.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: GeoJsonMap(), ); } } class GeoJsonMap extends StatefulWidget { @override _GeoJsonMapState createState() => _GeoJsonMapState(); } class _GeoJsonMapState extends State<GeoJsonMap> { List<Marker> _markers = []; @override void initState() { super.initState(); _loadMarkers(); } Future<void> _loadMarkers() async { List<GeoJsonPoint> points = await loadGeoJsonPoints(); List<Marker> markers = points.map((point) { return Marker( width: 80.0, height: 80.0, point: LatLng(point.geoPoint.latitude, point.geoPoint.longitude), builder: (ctx) => Container( child: Icon(Icons.location_on, color: Colors.red, size: 40.0), ), ); }).toList(); setState(() { _markers = markers; }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('GeoJSON Map')), body: FlutterMap( options: MapOptions( center: LatLng(0, 0), zoom: 2.0, ), layers: [ TileLayerOptions( urlTemplate: 'https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', subdomains: ['a', 'b', 'c'], ), MarkerLayerOptions(markers: _markers), ], ), ); } } |
Step 5: Run Your App
Now, run your Flutter app. You should see a map displaying markers at the specified locations in your GeoJSON file.
Conclusion
By following these steps, you can load a GeoJSON file and display markers on a map in your Flutter app. This approach leverages the flutter_map plugin for the map interface and the geojson package to handle the GeoJSON data. Adjust the map options and marker styles to fit your specific use case.
Don't miss out on this opportunity to hire Flutter developers and level up your app development skills!