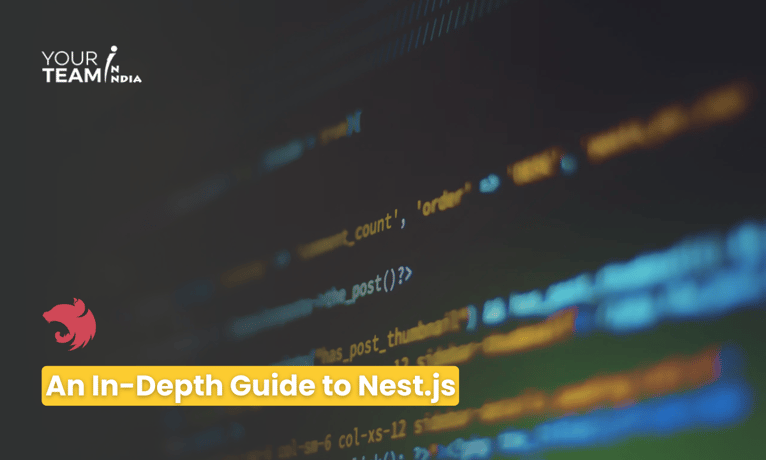
Quick Summary: Nest.js is a progressive Node.js framework for building efficient, reliable, and scalable server-side applications. It leverages TypeScript for robust development and integrates well with Angular. This guide explores its architecture, core concepts, and best practices to help you master Nest.js development.
Introduction
A progressive Node.js framework called Nest.js helps developers create dependable, scalable, and effective server-side apps. It uses TypeScript to provide decorators, strong typing, and other contemporary language features, all of which contribute to a robust development experience. Because Nest.js adheres to an Angular-inspired architectural design, it is incredibly modular and scalable.
Setting up Nest.js
You must have npm and Node.js installed in order to use Nest.js. You can use the Nest CLI to start a new Nest.js project:
By doing this, you may create a new Nest.js project and begin developing your application.
Controllers
In Nest.js, controllers are in charge of receiving requests and responding with the proper data. They act as the points of entry for the API endpoints in your application.
Creating a Controller
With the Nest CLI, a new controller can be created:
This will create a new ‘cats.controller.ts’ file in the ‘src’ directory.
In this example, we've defined a ‘CatsController’ with a single route ‘GET /cats’ that returns a string.
Providers:
An essential idea in Nest.js, providers are used to manage dependencies and organize code. These could be factories, services, repositories, or any other kind of object your application requires.
Creating a Service Provider
With the Nest CLI, you can create a new service provider:
This will create a new ‘cats.service.ts’ file in the ‘src’ directory.
In this example, we've defined a ‘CatsService’ with a ‘findAll’ method that returns an array of cats.
Modules
Modules in Nest.js are used to organize the application into cohesive blocks of functionality. They encapsulate related controllers, providers, and other code into reusable units.
Creating a Module
You can generate a new module using the Nest CLI:
This will create a new ‘cats.module.ts’ file in the ‘src’ directory.
In this example, we've defined a ‘CatsModule’ that imports the ‘CatsController’ and ‘CatsService’ and declares them as part of the module.
Middleware
Middleware in Nest.js are functions that have access to the request and response objects. They can modify the request or response, terminate the request-response cycle, or call the next middleware in the stack.
Creating Middleware
You can create custom middleware by defining a class with the @Injectable() decorator and implementing the NestMiddleware interface.
In this example, we've created a ‘LoggerMiddleware’ that logs a message for every incoming request.
Exception Filters
Exception filters in Nest.js are used to handle exceptions that occur during the execution of a request. They allow you to customize the error response sent back to the client.
Creating Exception Filters
You can create custom exception filters by defining a class with the ‘@Catch()’ decorator.
In this example, we've created an ‘HttpExceptionFilter’ that catches ‘HttpExceptions’ and sends back a JSON response with the exception message.
Pipes
Pipes in Nest.js are used to transform input data before it reaches the route handler or to validate the input data.
Creating Pipes
You can create custom pipes by defining a class with the ‘@Injectable()’ decorator and implementing the PipeTransform interface.
In this example, we've created a ‘ValidationPipe’ that can be used to transform or validate input data.
Guards
Guards in Nest.js are used to control access to routes based on certain conditions. They can be used for authentication, authorization, or any other type of access control.
Creating Guards
You can create custom guards by defining a class with the ‘@Injectable()’ decorator and implementing the CanActivate interface.
In this example, we've created an ‘AuthGuard’ that allows access to a route if the user is authenticated.
Interceptors
Interceptors in Nest.js are used to intercept incoming requests and outgoing responses. They allow you to modify the request or response before it reaches the route handler or the client.
Creating Interceptors
You can create custom interceptors by defining a class with the ‘@Injectable()’ decorator and implementing the NestInterceptor interface.
In this example, we've created a ’LoggingInterceptor’ that logs a message for every incoming request.
Custom Decorators
Nest.js allows you to create custom decorators to add metadata or functionality to your classes, methods, or properties.
Creating Custom Decorators
You can create custom decorators by defining a function that returns a decorator function.
In this example, we've created a 'User' decorator that extracts the user object from the request.
Advantages:
- Modular and Scalable Architecture: Nest.js encourages a modular structure that allows developers to organize code into modules, controllers, services, and more. This modularity fosters scalability as applications grow in complexity, enabling easy management and extension.
- TypeScript Support: TypeScript brings static typing to JavaScript, providing benefits such as enhanced code readability, better IDE support, and improved code quality through type checking. Nest.js leverages TypeScript to enable early error detection and better maintainability.
- Dependency Injection (DI): Nest.js employs a built-in dependency injection system that facilitates the management of dependencies and enhances code reusability. With DI, components can be easily injected into other components, promoting a modular and testable codebase.
- Built-in Support for WebSockets: Nest.js includes built-in support for WebSockets, allowing developers to create real-time communication features such as chat applications or live updates without relying on external libraries.
- Middleware Support: Middleware functions can be seamlessly integrated into the request processing pipeline in Nest.js, enabling the easy implementation and management of tasks such as authentication, logging, error handling, and more.
- Robust HTTP Exception Handling: Nest.js provides a comprehensive mechanism for handling HTTP exceptions, making it easier to manage errors and ensure a consistent response format across the application.
- Platform Agnostic: Nest.js is not tied to any specific HTTP server implementation, which means it can be deployed on various platforms like Express, Fastify, or even as a serverless function, giving developers flexibility in deployment options.
- Active Community and Ecosystem: Nest.js boasts a vibrant community and ecosystem, with a growing number of third-party modules, plugins, and integrations available. This active community provides valuable resources, support, and contributions to the framework.
- Well-Documented: Nest.js offers extensive documentation that covers various aspects of the framework, including concepts, features, best practices, and examples. This documentation serves as a valuable resource for both beginners and experienced developers.
- Testability: Nest.js promotes test-driven development (TDD) by providing built-in support for testing, including utilities for mocking dependencies, performing unit tests, and conducting end-to-end testing using tools like Jest.
Conclusion
Nest.js is a powerful framework for building efficient, scalable, and maintainable server-side applications with Node.js. It provides a robust architecture based on modules, controllers, and services, leveraging TypeScript's strong typing for enhanced productivity and maintainability.
With its built-in support for dependency injection, middleware, and decorators, Nest.js simplifies the development process, making it ideal for building complex applications while maintaining code organization and clarity. Overall, Nest.js offers developers a modern and feature-rich solution for building server-side applications in Node.js.
Get reliable, high-performance solutions. Hire Nest.js developers to enhance your team!