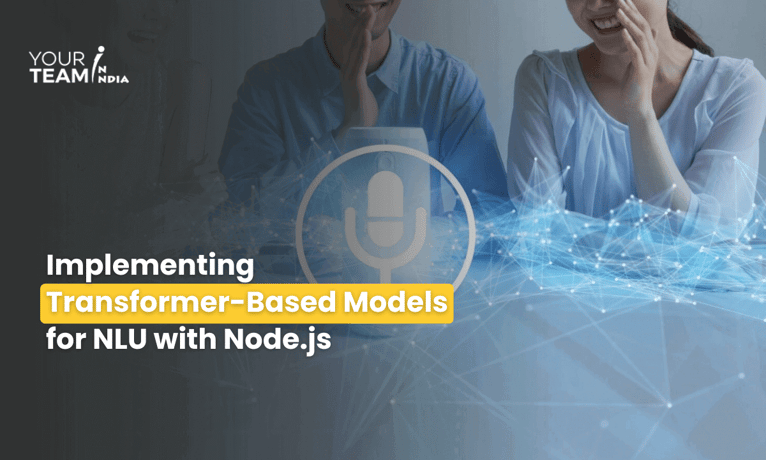
Quick Summary: In Natural Language Understanding, harnessing the power of Transformer-Based Models is a game-changer. This article delves into the exciting world of NLP and demonstrates how to leverage the capabilities of Transformer-Based Models with Node.js, opening doors to enhanced language processing and understanding.
Introduction
Natural Language Understanding (NLU) is a crucial aspect of many AI applications, including chatbots, sentiment analysis, language translation, and more. Transformer-based models have revolutionized the field of NLU, offering state-of-the-art performance on a wide range of tasks. In this article, we'll explore how to implement Transformer-based models for NLU using Node.js.
What is a Transformer-Based Model?
Transformer-based models, introduced in the paper "Attention is All You Need" by Vaswani et al., have become the foundation of modern NLU tasks. They excel in handling sequential data, making them perfect for understanding and generating human language. Key features of Transformer models include self-attention mechanisms, parallelization, and the ability to capture long-range dependencies.
Setting Up the Environment
Before diving into the code, let's ensure we have the necessary tools and libraries installed:
- Node.js: Make sure you have Node.js installed on your system. You can download it from nodejs.org.
- npm: Node.js comes with npm (Node Package Manager) by default.
- Transformers Library: We'll use the "transformers" library for Node.js, which provides pre-trained Transformer models and a straightforward API for using them. Install it using:
npm install transformers |
4. TensorFlow.js (Optional): If you want to run models in the browser or on Node.js using TensorFlow.js, install it as well:
npm install @tensorflow/tfjs-node |
Loading a Pre-trained Transformer Model
Let's load a pre-trained Transformer model for NLU tasks using the "transformers" library. We'll use the Hugging Face Transformers library, which offers various pre-trained models for NLU.
const { AutoModelForSequenceClassification, AutoTokenizer } = require("transformers"); // Load a pre-trained model and tokenizer const model = await AutoModelForSequenceClassification.fromPretrained("bert-base-uncased"); const tokenizer = await AutoTokenizer.fromPretrained("bert-base-uncased"); |
In this example, we're loading the "bert-base-uncased" model, which is pre-trained on a large corpus of text and is suitable for various NLU tasks.
Using the Transformer Model for Text Classification
Now, let's use the loaded model and tokenizer to perform text classification on a sample text.
use the Hugging Face Transformers library, which offers various pre-trained models for NLU.
const text = "I love using Transformer models for NLU!"; const labels = ["Negative", "Positive"]; // Replace with your task-specific labels // Tokenize the text const inputs = tokenizer.encode(text, { truncation: true, padding: "max_length", max_length: 64, return_tensors: "pt" }); // Perform inference const logits = model(inputs.input_ids, inputs.attention_mask); const probabilities = logits.softmax(dim = 1); // Get the predicted label const predictedLabelIndex = probabilities.argmax(); const predictedLabel = labels[predictedLabelIndex]; console.log(`Predicted Label: ${predictedLabel}`); |
In this code snippet, we tokenize the input text, pass it through the model, and obtain the predicted label. Make sure to replace the labels array with the specific labels relevant to your classification task.
Running in the Browser or on Node.js
If you want to run the model in a browser or on Node.js using TensorFlow.js, you can convert the model using the following code:
const tf = require("@tensorflow/tfjs-node"); const tfModel = await tf.loadLayersModel("https://huggingface.co/bert-base-uncased-tfjs/model.json"); |
Now, you can use tfModel for inference in TensorFlow.js.
Conclusion
This article explored implementing Transformer-based models for Natural Language Understanding using Node.js. We loaded a pre-trained model, tokenized text, and performed text classification. These models can be fine-tuned for various NLU tasks and integrated into your AI applications, making them more capable of understanding human language. Remember that the "transformers" library provides a wide range of pre-trained models, allowing you to choose the one that best suits your specific NLU task.
Ready to Build Your Node.js App? Elevate efficiency and reduce your development costs by hiring Node.js developers from Your Team in India.