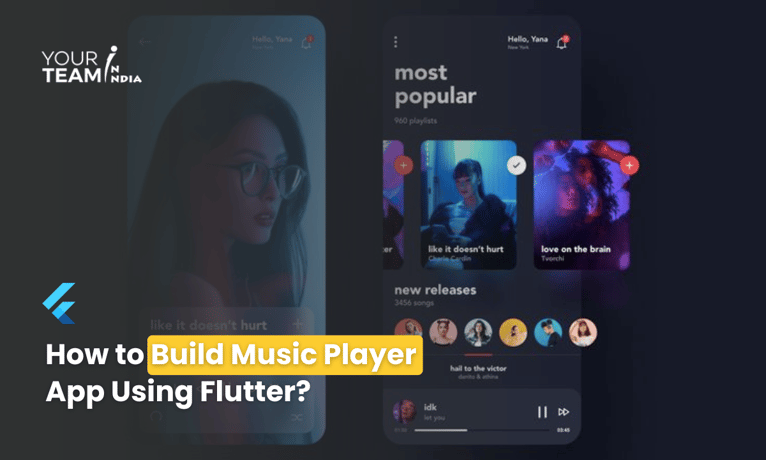
Quick Summary: Embark on creating a feature-rich music player application using Flutter with this step-by-step guide. Learn the essential components, libraries, and best practices to build a seamless, responsive, and user-friendly music player app from scratch.
Introduction
- The popularity of music player apps and their demand in the mobile app market.
- Overview of the Flutter framework for cross-platform app development.
- The goal is to build a feature-rich music player app using Flutter.
Setting Up the Flutter Project
- Creating a New Flutter Project
- Using the Flutter CLI to create a new project.
- Configuring project settings.
flutter create music_player_app |
Adding Dependencies
- Integrating necessary dependencies for building a music player app.
- Dependencies for audio playback, UI components, and state management.
# pubspec.yaml |
flutter pub get |
Designing the Music Player UI
- Creating the App Layout
- Designing the main layout for the music player app.
- Organizing components such as play controls, album artwork, and track information.
- Implementing Navigation
- Setting up navigation between screens (e.g., playlist view, now playing view).
- Utilizing Flutter's navigation system.
Integrating Audio Playback
- Using the Audioplayers Package
- Initializing the audio player instance.
- Handling audio playback controls (play, pause, skip).
// Example: Initializing audioplayers |
Updating UI Based on Playback State
- Reflecting the current playback state in the UI.
- Implementing callbacks for play/pause button actions.
// Example: Updating UI based on playback state |
Managing State with Provider
- Using Provider for State Management
- Integrating the Provider package for managing app state.
- Creating a centralized state management solution.
// Example: Using Provider for state management |
Updating UI with Provider
- Utilizing the Provider.of method to update UI elements based on state changes.
- Ensuring a reactive and efficient UI.
// Example: Updating UI with Provider |
Implementing Playlist Features
- Displaying Playlists
- Fetching and displaying a list of available playlists.
- Creating a user-friendly interface for selecting playlists.
- Managing Playlist State
- Implementing features for adding, removing, and organizing playlists.
- Utilizing state management to handle playlist-related actions.
Enhancing the User Experience
- Adding Visual Effects
- Incorporating animations and transitions for a polished UI.
- Utilizing Flutter's animation framework.
- Implementing Themes and Styling
- Allowing users to customize the app's appearance.
- Implementing a theme switcher for light and dark modes.
Testing and Debugging
- Testing the Music Player App
- Writing unit tests for critical app functionalities.
- Ensuring a smooth and bug-free user experience.
- Debugging and Performance Optimization
- Using Flutter's debugging tools for identifying and fixing issues.
- Optimizing the app for performance and responsiveness.
Let's focus on the initial setup and creating the basic UI for the music player app. This example assumes you have the necessary dependencies installed.
Step 1: Set Up the Flutter Project
Use the following commands to create a new Flutter project and add the required dependencies:
flutter create music_player_app |
Add dependencies to your pubspec.yaml file:
dependencies: |
Run flutter pub get to install the dependencies.
Step 2: Create the Main App and UI
Create a file named main.dart in the lib directory and add the following code:
import 'package:flutter/material.dart'; |
In this example:
-
We've created a MusicPlayerState class using ChangeNotifier to manage the audio player state.
-
The MyApp widget uses ChangeNotifierProvider to provide the MusicPlayerState to the entire app.
-
The MyHomePage widget displays a basic UI with a text label and a play/pause button.
-
The play/pause button's functionality is tied to the togglePlayback method in the MusicPlayerState.
Remember to replace 'path/to/your/audio.mp3' with the actual path to your audio file. This example sets up the foundation for the music player app, and you can expand upon it by adding more features, screens, and interactions based on your app's requirements.
Conclusion
- Recap of key steps in building a music player app with Flutter.
- Encourage developers to explore additional features and customization options.
- Suggestions for potential app enhancements and future development.
Get free consultation from the best flutter development company in india to elevate your Flutter app design. Unlock the full potential of Flutter layouts with our professional Flutter developers.