Quick Summary: Explore the powerful combination of Flutter and machine learning with TensorFlow Lite integration in this comprehensive guide. Learn how to seamlessly incorporate TensorFlow Lite into your Flutter applications to build intelligent, real-time, and on-device machine learning features for enhanced user experiences.

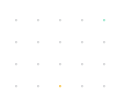
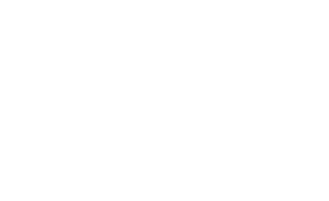
Want to build your Flutter App?
Discover the power of seamless performance, responsive UI, and cross-platform compatibility with Flutter Apps.
Introduction
- Brief overview of the intersection of Flutter and machine learning.
- Introduction to TensorFlow Lite as a lightweight machine learning framework.
- The benefits of integrating TensorFlow Lite with Flutter for on-device machine learning.
Understanding TensorFlow Lite
- What is TensorFlow Lite?
- Explanation of TensorFlow Lite as a lightweight and mobile-friendly version of TensorFlow.
- Overview of its role in deploying machine learning models on mobile and edge devices.
- Supported Models and Formats
- List of supported machine learning models and formats in TensorFlow Lite.
- Understanding the compatibility with popular model types (e.g., TensorFlow, Keras).
Integrating TensorFlow Lite with Flutter
- Adding TensorFlow Lite Dependency
- Adding the TensorFlow Lite plugin to the Flutter project.
- Configuring the pubspec.yaml file.
# pubspec.yaml |
// Importing TensorFlow Lite package |
Loading a TensorFlow Lite Model
- Loading a pre-trained machine learning model into a Flutter app.
- Ensuring the model is compatible with TensorFlow Lite.
// Example: Loading a TensorFlow Lite model |
Running Inference with TensorFlow Lite
- Processing Input Data
- Preparing input data for the TensorFlow Lite model.
- Ensuring input data is formatted correctly for inference.
// Example: Processing input data |
Interpreting Output Results
- Interpreting the output results from the TensorFlow Lite model.
- Understanding the meaning of the output data.
// Example: Interpreting output results |
Integrating with Flutter UI
- Displaying Model Results
- Displaying the results of machine learning inference in the Flutter user interface.
- Building visualizations or providing user-friendly feedback.
// Example: Displaying model results in Flutter UI |
Real-time Inference |
Handling Model Updates
- Updating TensorFlow Lite Models
- Strategies for handling model updates and retraining.
- Ensuring smooth updates without disrupting the app.
// Example: Updating TensorFlow Lite model |
Retraining Models
- Overview of the process of retraining machine learning models.
- Incorporating retrained models into Flutter apps.
// Example: Retraining TensorFlow Lite model |
Testing and Debugging
- Testing TensorFlow Lite Integration Locally
- Configuring a testing environment for TensorFlow Lite in Flutter.
- Utilizing sample data for testing inference.
# Example: Testing TensorFlow Lite locally |
Debugging TensorFlow Lite Issues
- Techniques for debugging common issues with TensorFlow Lite integration.
- Utilizing Flutter's debugging tools for machine learning inference.
flutter run --enable-software-rendering |
Conclusion
- Recap of key steps in integrating TensorFlow Lite with Flutter for machine learning.
- Encouragement for developers to explore various use cases for on-device machine learning.
- Reminders about the importance of testing and optimizing for performance in machine learning integration.
Get free consultation from the best flutter development company in india to elevate your Flutter app design. Unlock the full potential of Flutter layouts with our professional Flutter developers.