Quick Summary: This blog post explores the integration of Flutter and Cloud Functions to create scalable and efficient serverless mobile applications. It covers the benefits of this approach, including reduced development time, cost-effectiveness, and improved scalability.

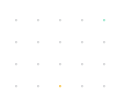
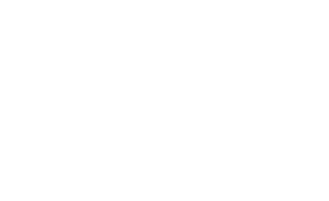
Build Your App with Flutter and Cloud Functions
Our team of experts specializes in Flutter and Cloud Functions, delivering exceptional results.
Introduction
What is Flutter?
Flutter is an open-source UI toolkit developed by Google for creating natively compiled applications for mobile, web, and desktop from a single codebase. Leveraging the Dart programming language, Flutter is known for its high performance, rich widget library, and rapid development capabilities.
What are Cloud Functions?
Cloud Functions are a serverless compute service provided by cloud platforms like Google Cloud, AWS, and Azure. They allow developers to run backend code in response to events without managing servers. Cloud Functions automatically scale based on demand, and you only pay for the compute time used.
Key Features:
- Event-Driven: Executes code in response to events (e.g., HTTP requests, database changes).
- Scalability: Automatically scales up or down based on traffic.
- No Server Management: Focus solely on code without worrying about server infrastructure.
Cost Efficiency: Pay only for the execution time and resources consumed.
Integrating Cloud Functions with Flutter
Discover the step-by-step guide to integrating Cloud Functions into your Flutter projects. To fully harness the power of Flutter and Cloud Functions, consider partnering with experienced Flutter app development company. Let's dive into the code:
1. Add Dependencies
In your Flutter project, you may need to add dependencies for HTTP requests. For example, in pubspec.yaml:
dependencies: flutter: sdk: flutter http: ^0.14.0 |
Run flutter pub get to install the dependency.
2. Call Cloud Functions from Flutter
Use the http package to make HTTP requests to your Cloud Function. Here’s an example of how to call the function from a Flutter app:
import 'package:flutter/material.dart'; import 'package:http/http.dart' as http; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar(title: Text('Cloud Functions with Flutter')), body: Center( child: ElevatedButton( onPressed: _callCloudFunction, child: Text('Call Cloud Function'), ), ), ), ); } void _callCloudFunction() async { final response = await http.get(Uri.parse('https://REGION-PROJECT_ID.cloudfunctions.net/helloWorld')); if (response.statusCode == 200) { print('Response: ${response.body}'); } else { print('Failed to call Cloud Function'); } } |
Setting Up Cloud Functions
1. Choose a Cloud Provider
Common providers include Google Cloud Functions, AWS Lambda, and Azure Functions. For this guide, we’ll use Google Cloud Functions.
2. Set Up Your Cloud Functions Environment
- Install Google Cloud SDK: Follow the installation guide at Google Cloud SDK.
- Authenticate: Run gcloud auth login to authenticate your Google Cloud account.
- Set Up Project: Create a Google Cloud project and enable the Cloud Functions API.
3. Write Your Cloud Function
Create a Cloud Function using your preferred language (Node.js, Python, etc.). Here’s a simple example in Node.js that responds to HTTP requests:
const functions = require('@google-cloud/functions-framework'); functions.http('helloWorld', (req, res) => { res.send('Hello, World!'); }); |
Deploy this function using the Google Cloud CLI:
gcloud functions deploy helloWorld --runtime nodejs14 --trigger-http --allow-unauthenticated |
4. Test Your Cloud Function
Once deployed, you can test your function using the provided URL. For example:
curl https://REGION-PROJECT_ID.cloudfunctions.net/helloWorld |
Need help integrating Cloud Functions with your Flutter app? Our team of experienced Flutter developers can provide expert guidance and assistance.
Use Cases
- Backend Logic: Handle business logic that is too complex or sensitive to be handled directly on the client side.
- Data Processing: Perform tasks like image processing, data validation, or transformation.
- Third-Party Integrations: Connect to external services or APIs securely.
- Real-Time Updates: Respond to changes in databases or other services.
Benefits of Using Cloud Functions with Flutter
- Serverless Architecture: Reduces server management overhead and scales automatically.
- Cost-Effective: Pay only for the execution time, not for idle server time.
- Flexibility: Easily integrates with various cloud services and APIs.
- Improved Security: Sensitive operations and data processing occur on the server side, not on the client.
Conclusion
Combining Flutter with Cloud Functions offers a powerful approach to mobile app development by leveraging serverless architecture. This integration enables developers to offload complex or sensitive operations to the backend while maintaining a seamless and responsive user experience in the Flutter app. By focusing on business logic and functionality rather than infrastructure management, developers can build scalable, efficient, and secure mobile applications.