Quick Summary: This blog post explores the integration of Flutter and Amazon S3 for efficient file uploading and retrieval in mobile applications. It covers the benefits of using Amazon S3 for file storage, the steps involved in setting up and configuring S3 in a Flutter app, and the process of uploading and downloading files.
Introduction
What is Flutter?
Flutter is an open-source UI toolkit developed by Google for creating natively compiled applications for mobile, web, and desktop from a single codebase. It uses the Dart programming language and provides a rich set of pre-designed widgets to build high-performance, visually appealing apps.
What is Amazon S3?
Amazon Simple Storage Service (S3) is a scalable object storage service provided by AWS (Amazon Web Services). It allows you to store and retrieve any amount of data at any time, making it ideal for handling files such as images, videos, and documents.
Integrating Flutter with Amazon S3
To upload and retrieve files from Amazon S3 in a Flutter application, you typically use the AWS SDK or third-party packages that facilitate interactions with S3. This guide provides a step-by-step approach to achieve this integration.
Key Steps for Integration
1. Set Up Your Amazon S3 Bucket
Create an S3 Bucket:
- Go to the AWS Management Console.
- Navigate to S3 and create a new bucket.
- Configure bucket settings such as permissions and region.
2. Get AWS Credentials:
Obtain your Access Key ID and Secret Access Key from the AWS IAM console. Create an IAM user with permissions to access S3.
3. Add Dependencies to Flutter
Use the aws_sdk_flutter or flutter_aws_s3_client package for S3 operations. Update your pubspec.yaml:
dependencies: flutter: sdk: flutter aws_sdk_flutter: ^0.1.0 # Check for the latest version # or flutter_aws_s3_client: ^0.2.0 # Check for the latest version |
Run flutter pub get to install the package.
3. Configure AWS SDK in Flutter
Initialize the AWS SDK in your Flutter application. For the aws_sdk_flutter package, configure it as follows:
import 'package:aws_sdk_flutter/aws_sdk_flutter.dart'; void main() async { WidgetsFlutterBinding.ensureInitialized(); final awsClient = AwsClient( accessKey: 'YOUR_ACCESS_KEY', secretKey: 'YOUR_SECRET_KEY', region: 'YOUR_REGION', ); runApp(MyApp(awsClient: awsClient)); } |
For flutter_aws_s3_client, you can use it directly to interact with S3.
4. Upload Files to S3
Use the flutter_aws_s3_client package to upload files. Here’s an example of uploading a file:
import 'package:flutter/material.dart'; import 'package:flutter_aws_s3_client/flutter_aws_s3_client.dart'; class UploadFile extends StatefulWidget { @override _UploadFileState createState() => _UploadFileState(); } class _UploadFileState extends State<UploadFile> { final _s3Client = FlutterAwsS3Client( region: 'YOUR_REGION', bucketId: 'YOUR_BUCKET_NAME', accessKey: 'YOUR_ACCESS_KEY', secretKey: 'YOUR_SECRET_KEY', ); Future<void> _uploadFile() async { try { final file = // Obtain the file you want to upload final result = await _s3Client.uploadFile( file: file, key: 'file-key', ); print('Upload successful: $result'); } catch (e) { print('Error uploading file: $e'); } } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Upload File')), body: Center( child: ElevatedButton( onPressed: _uploadFile, child: Text('Upload File'), ), ), ); } } |
5. Retrieve Files from S3
To retrieve files, you can use the following example;
import 'package:flutter/material.dart'; import 'package:flutter_aws_s3_client/flutter_aws_s3_client.dart'; class RetrieveFile extends StatefulWidget { @override _RetrieveFileState createState() => _RetrieveFileState(); } class _RetrieveFileState extends State<RetrieveFile> { final _s3Client = FlutterAwsS3Client( region: 'YOUR_REGION', bucketId: 'YOUR_BUCKET_NAME', accessKey: 'YOUR_ACCESS_KEY', secretKey: 'YOUR_SECRET_KEY', ); Future<void> _retrieveFile() async { try { final file = await _s3Client.getFile(key: 'file-key'); print('File retrieved: ${file.uri}'); } catch (e) { print('Error retrieving file: $e'); } } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Retrieve File')), body: Center( child: ElevatedButton( onPressed: _retrieveFile, child: Text('Retrieve File'), ), ), ); } } |
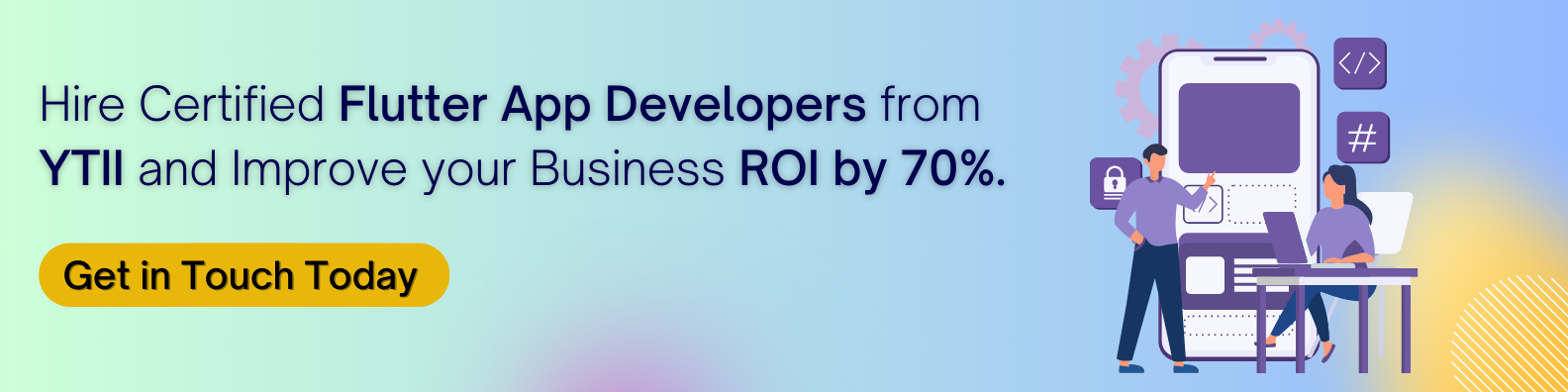
Summary:
- Set Up S3 Bucket: Create and configure an S3 bucket in AWS.
- Add Dependencies: Install the necessary Flutter packages for S3 integration.
- Configure AWS SDK: Initialize AWS credentials and configure the SDK
- Upload Files: Use the S3 client to upload files to your S3 bucket.
- Retrieve Files: Use the S3 client to download or access files from S3.
Integrating Amazon S3 with Flutter allows you to leverage cloud storage for handling file uploads and retrievals, enabling scalable and efficient management of your application’s data assets. Hire Flutter Developers for expert assistance in integrating S3 with your Flutter app and optimizing its performance.