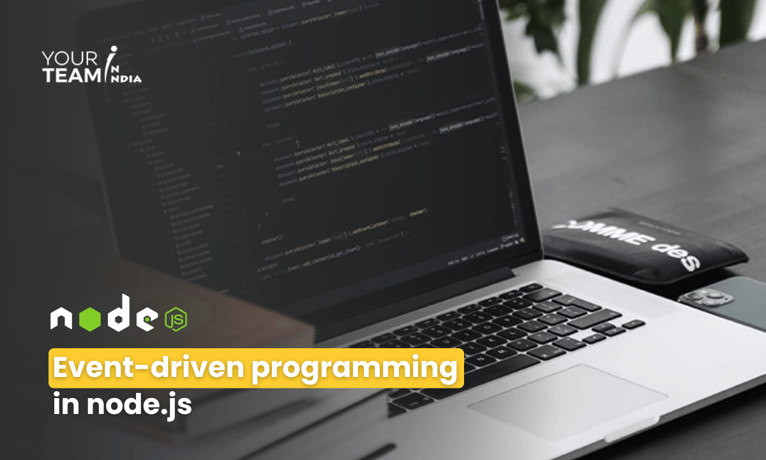
Quick Summary: Event-driven programming in Node.js enables efficient, non-blocking execution by handling asynchronous operations with events and callbacks. This approach is crucial for building scalable, high-performance applications that can handle numerous simultaneous connections, making Node.js a popular choice for real-time applications and microservices.
Introduction
Event-driven programming is a paradigm that revolves around the occurrence of events. This programming model is particularly well-suited for building applications that require asynchronous behavior, such as web servers, GUIs, and real-time applications. Node.js, with its non-blocking I/O and event-driven architecture, is a powerful platform for developing such applications. In this article, we will explore the principles of event-driven programming in Node.js, from the basics to advanced concepts, and conclude with practical examples to solidify our understanding.
1. Understanding Event-Driven Programming
Event-driven programming is centered around the concept of events and event handlers. An event is an action or occurrence recognized by software, and an event handler (or listener) is a callback function that is executed in response to an event. This model decouples the code that emits events from the code that handles them, promoting modularity and flexibility.
Key Concepts:
- Events: Actions or occurrences, such as user interactions, sensor readings, or messages.
- Event Loop: A mechanism that listens for and dispatches events.
- Event Emitters: Objects that generate events. In Node.js, the EventEmitter class is the core for creating event-driven applications.
2. Event-Driven Architecture in Node.js
Node.js is built on the V8 JavaScript engine and uses an event-driven, non-blocking I/O model. This architecture allows Node.js to handle multiple connections simultaneously without the need for multi-threading.
Key Components:
- Event Loop: The heart of Node.js's event-driven architecture. It continuously checks for events and dispatches them to the appropriate handlers.
- EventEmitter Class: Provides a mechanism to create and handle custom events.
3. Working with EventEmitter
The EventEmitter class in Node.js is a powerful tool for building event-driven applications. It allows developers to define custom events and assign handlers to them.
Basic Usage:
Advanced Usage:
- Passing Arguments: Handlers can receive arguments when an event is emitted.
- Once Handlers: Handlers that execute only once.
4. Asynchronous Programming with Events
One of the strengths of Node.js is its ability to handle asynchronous operations using events. This is essential for building scalable and performant applications.
Example: Reading a File
In this example, fs.readFile emits an event when the file read operation completes, and the callback function handles the event.
5. Real-world Applications
Web Servers
Node.js excels at building web servers due to its event-driven architecture.
Real-Time Applications
Applications such as chat servers, gaming servers, and live data streaming services benefit greatly from Node.js's event-driven model.
Advantages
- Asynchronous and Non-Blocking I/O:
- Node.js's event-driven architecture allows for non-blocking I/O operations, which means that tasks can be executed in the background while other tasks continue to run. This leads to efficient use of resources and improved performance, especially in I/O-heavy applications like web servers and APIs.
- Scalability:
- Event-driven programming in Node.js can handle a large number of concurrent connections with minimal resource consumption. This makes it ideal for building scalable network applications, such as real-time chat applications, gaming servers, and live data feeds.
- Responsiveness:
- The non-blocking nature of event-driven programming ensures that applications remain responsive, even when performing intensive operations. User interfaces can remain active and responsive to user actions, improving the overall user experience.
- Simplicity and Code Modularity:
- By decoupling event emitters from event handlers, the codebase becomes more modular and easier to manage. Each event handler can be defined separately, promoting better organization and maintainability of the code.
- Resource Efficiency:
- Node.js's single-threaded event loop can efficiently manage memory and CPU usage, reducing the overhead associated with multi-threading. This makes Node.js suitable for applications running on limited-resource environments, such as cloud services or IoT devices.
- Community and Ecosystem:
- The Node.js ecosystem includes a vast number of modules and libraries that leverage event-driven programming. This extensive ecosystem allows developers to integrate various functionalities quickly and accelerates the development process.
Disadvantages
- Complexity in Error Handling:
- Asynchronous code and callbacks can lead to complex error-handling scenarios. Managing errors in a callback-based approach can become cumbersome, often referred to as "callback hell." While Promises and async/await syntax have mitigated this issue, developers must still be cautious with error propagation.
- Debugging Challenges:
- Debugging asynchronous code can be more challenging compared to synchronous code. Tracing the flow of events and understanding the sequence of asynchronous operations can be difficult, especially in complex applications.
- Single Thread Limitation:
- Although Node.js is efficient with its single-threaded event loop, CPU-bound tasks can become bottlenecks. Node.js is not well-suited for applications that require heavy computation, such as data processing or scientific calculations. These tasks can block the event loop and degrade performance.
- Callback Hell:
- Without careful design, event-driven programming can lead to deeply nested callbacks, known as "callback hell," which makes the code difficult to read and maintain. Promises and async/await syntax have alleviated this issue, but it remains a potential pitfall for less experienced developers.
- Concurrency Control:
- While Node.js can handle many concurrent connections, managing shared resources and ensuring proper synchronization between asynchronous operations can be challenging. Developers must use techniques such as mutexes or other concurrency control mechanisms to avoid race conditions and data inconsistencies.
- Learning Curve:
- For developers coming from synchronous programming backgrounds, adopting an event-driven approach can require a significant shift in thinking. Understanding the nuances of asynchronous programming, event loops, and callback management can take time and practice.
Conclusion
Event-driven programming in Node.js offers significant advantages such as asynchronous I/O, scalability, and responsiveness. However, it comes with challenges like complex error handling and debugging difficulties. By mastering its principles and utilizing proper design patterns, developers can leverage Node.js's event-driven model to build high-performance applications efficiently.
Ready to Build Your Node.js App? Elevate efficiency and reduce your development costs by hiring developers from the best Nodejs development company in India.