Quick Summary: Discover how to build high-performance Jamstack applications using Next.js. This article explores the benefits of combining static site generation, serverless functions, and modern web technologies, ensuring speed, scalability, and seamless user experiences. Learn best practices, tools, and tips to create dynamic and efficient Jamstack applications tailored to your needs.

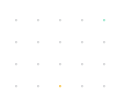
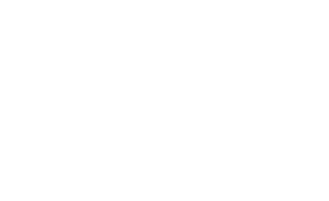
Elevate your projects and deliver outstanding user experiences.
Transform your web development journey with our skilled Next.js developers.
In the rapidly evolving web development landscape, Jamstack has emerged as a revolutionary architecture that prioritizes speed, scalability, and a better developer experience. The term “Jamstack” stands for JavaScript, APIs, and Markup, signifying a modern approach to building web applications. Unlike traditional monolithic systems, Jamstack decouples the front end and back end, enabling developers to build lightning-fast applications with enhanced security.
At the heart of this architecture is Next.js, a powerful React-based framework that makes it easier to create Jamstack applications. With its ability to render pages both statically and dynamically, support for API routes, and seamless integration with headless CMS systems, Next.js has become a go-to tool for modern web developers.
Key Features of Jamstack
Jamstack is characterized by three core principles:
- Pre-rendering: Pages are generated during build time and serve as static files, ensuring rapid delivery.
- Decoupled Architecture: The frontend is separate from the backend, allowing you to integrate multiple services.
- CDN-based Delivery: Content is served from Content Delivery Networks (CDNs), reducing latency and increasing speed.
These principles collectively make Jamstack applications faster, more secure, and easier to scale.
Why Choose Next.js for Jamstack?
Next.js has proven itself as a robust framework tailored to Jamstack’s philosophy. Here’s why it stands out:
1. Hybrid Rendering
Next.js supports both Static Site Generation (SSG) and Server-Side Rendering (SSR). While SSG aligns perfectly with Jamstack’s goal of pre-rendered content, SSR is useful when dynamic data needs to be fetched at runtime.
2. API Routes
With Next.js, you can create serverless functions directly within the project. These functions act as APIs and eliminate the need for a dedicated backend server, keeping the architecture lightweight.
3. Automatic Code Splitting
Next.js automatically splits your code into smaller bundles, delivering only the necessary code to the browser. This improves performance and speeds up page loading times.
4. Headless CMS Compatibility
Next.js integrates seamlessly with headless CMS platforms like Contentful, Strapi, and Sanity. These CMS platforms allow developers to manage content independently from the presentation layer.
5. Built-in Optimization
Optimizing images and JavaScript files in Next.js is effortless. Features like Image Optimization and Script Loading improve performance without manual intervention.
Building a Jamstack Application With Next.js
Let’s walk through the steps to build a basic Jamstack application using Next.js.
Step 1: Setting Up the Environment
Start by creating a new Next.js application:
bash
Copy code
npx create-next-app@latest jamstack-app
cd jamstack-app
This sets up the project with all the necessary dependencies.
Step 2: Create Static Pages
In Jamstack, most pages are pre-rendered as static files. You can create a static homepage like this:
javascript
Copy code
// pages/index.js
export default function Home() {
return (
<div>
<h1>Welcome to My Jamstack App</h1>
<p>This is a static page served from a CDN.</p>
</div>
);
}
Step 3: Add Dynamic Data With getStaticProps
For dynamic content, fetch data during the build process:
javascript
Copy code
// pages/blog.js
export async function getStaticProps() {
const res = await fetch('https://api.example.com/posts');
const posts = await res.json();
return { props: { posts } };
}
export default function Blog({ posts }) {
return (
<div>
<h1>Blog Posts</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
}
Step 4: Configure API Routes
Add a simple API endpoint for your application:
javascript
Copy code
// pages/api/hello.js
export default function handler(req, res) {
res.status(200).json({ message: 'Hello, Jamstack!' });
}
Step 5: Deploy on a Jamstack Platform
Deploy your application on platforms like Vercel or Netlify. These platforms optimize static content delivery through CDNs. To deploy on Vercel:
bash
Copy code
npx vercel
Follow the prompts, and your application will be live in seconds.
Benefits of Using Jamstack With Next.js
1. Enhanced Performance
With pre-rendered static content, Jamstack applications load faster, offering an improved user experience.
2. Scalability
CDN-first delivery ensures your application can handle high traffic without server strain.
3. Improved Security
With no direct backend to exploit, Jamstack applications are inherently more secure.
4. Developer Productivity
The combination of Next.js and Jamstack reduces complexity, allowing developers to focus on creating rich user experiences.
Use Cases of Jamstack Applications
- E-commerce Websites: Create fast-loading and secure online stores.
- Marketing Websites: Build promotional websites that are optimized for SEO.
- Blogs and Portfolios: Use static generation for quick delivery and content management.
- Dashboards: Integrate dynamic APIs for real-time data while keeping the front end decoupled.
Conclusion
Building Jamstack applications with Next.js is a game-changer for modern web development. By leveraging the framework's hybrid rendering capabilities, API routes, and built-in optimization, developers can create applications that are fast, secure, and highly scalable. As more businesses prioritize performance and user experience, the adoption of Jamstack with Next.js is expected to grow exponentially.
Start building your Jamstack application today with Next.js, and unlock the future of web development!
Looking to build scalable, high-performance web applications? Hire our expert Next.js developers to bring your vision to life with speed and precision.