Quick Summary: This guide provides a step-by-step tutorial on integrating Bluedot’s geolocation services into Android apps. By following this complete guide, developers can implement geofencing and location-based features, enhancing app functionality and delivering precise, location-aware experiences to users.

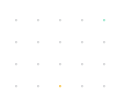
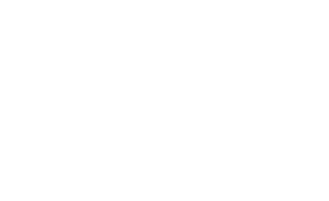
Hire Android App Developers
Ready to transform concepts into dynamic applications? Fuel your Android app ambitions with our expert developers!
Bluedot offers a powerful geolocation platform that enables developers to build location-based services in their Android applications with precision. In this comprehensive guide, we will walk you through integrating Bluedot into your Android app, from setting up the SDK to implementing advanced geofencing and location-aware featujres.
Whether you are creating apps for navigation, logistics, or personalized marketing, Bluedot provides the tools to leverage geolocation seamlessly. This tutorial will equip you with the knowledge and steps necessary to enhance user engagement through precise location detection and triggers. Let’s dive into the world of Bluedot for Android development!
Bluedot Android Tutorial
Bluedot is a mobile location awareness platform that provides high-accuracy, always-on location awareness and low-energy capabilities to mobile apps. It can be used to create location-based experiences such as geo-fencing, geo triggering, and indoor navigation.
Hire Seasoned Android App Developers
Looking to bring your Android projects to life? Our team of skilled Android developers is ready to turn your ideas into reality.
Import the SDK
Edit the root build.gradle file
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Include the Bluedot Point SDK as a dependency in the app’s build.gradle file
dependencies {
implementation 'com.gitlab.bluedotio.android:point_sdk_android:15.5.3'
}
Manifest requirements
In order to receive Bluedot service error events after initialization, a class that implements BluedotServiceReceiver should be implemented and registered in the AndroidManifest:
class ExampleBluedotServiceReceiver : BluedotServiceReceiver() {
override fun onBluedotServiceError(error: BDError, context: Context) {
// Handle error here.
}
}
<application android:label="@string/app_name" >
<receiver
android:name="<path to your BluedotServiceReceiverimplementation>"
android:enabled="true"
android:exported="false">
<intent-filter>
<action android:name="io.bluedot.point.SERVICE" />
</intent-filter>
</receiver>
</application>
<uses-permission
android:name="android.permission.ACCESS_BACKGROUND_LOCATION"
tools:node="remove"
/>
Initialize the SDK
Now that the project has been set up, should initialize the Bluedot Point SDK from your Application class’s onCreate function with:
ServiceManager.getInstance(this).initialize("myProjectId",
(error) -> {
// Handle initialization result
});
Start Geo-triggering
Geo-triggering allows the automatic detection of location context change events (such as entering or exiting a geofence, or crossing a Geoline™).
if (ServiceManager.getInstance(context).isBluedotServiceInitialized()) {
// The Bluedot SDK is initialized, you can start Geo-triggering.
GeoTriggeringService.builder()
.notification(notificationReference) // Notification to use to run Geo-triggering as a foreground service.
.notificationId(myNotificationId) // Optional id to use for foreground service notification. Use if your app will
// run additional foreground services, or you wish to update the notification.
.start(myApplicationContext, // This context should be the Application context.
error -> {
if (error != null) {
// Something went wrong when starting Geo-triggering. Handle error here.
return;
}
// Geo-triggering has started successfully. Handle success here.
});
} else {
// The Bluedot SDK is not initialized. Initialize before starting Geo-triggering.
}
Receiving Geo-trigger events
<application android:label="@string/app_name" >
<receiver
android:name="my.package.ExampleGeoTriggerReceiver"
android:enabled="true"
android:exported="false">
<intent-filter>
<action android:name="io.bluedot.point.GEOTRIGGER" />
</intent-filter>
</receiver>
</application>
class ExampleGeoTriggerReceiver : GeoTriggeringEventReceiver() {
override fun onZoneInfoUpdate(zones: List<ZoneInfo>, context: Context) {
// Notification that the local cache of zones has been updated.
}
override fun onZoneEntryEvent(entryEvent: ZoneEntryEvent, context: Context) {
// Notification that a zone has been entered/Geoline™ crossed.
}
override fun onZoneExitEvent(exitEvent: ZoneExitEvent, context: Context) {
// Notification that an exit detection-enabled zone has been exited.
}
}
Stop Geo-triggering
GeoTriggeringService.stop(myContext, error -> {
if (error != null) {
// Something went wrong stopping Geo-triggering. Handle error here.
return;
}
// Geo-triggering stopped successfully. Handle success here.
});
Conclusion
In conclusion, integrating Bluedot’s geolocation services into your Android app allows you to harness the power of precise location-based features. This tutorial has guided you through the steps of setting up the SDK, implementing geofencing, and customizing location triggers.
With Bluedot’s robust platform, you can create dynamic, context-aware apps that respond in real-time to user locations, enhancing engagement and functionality. Whether for navigation, logistics, or personalized marketing, Bluedot offers powerful tools to improve your app’s performance. Explore further to unlock more features and optimize your app’s location-based capabilities.