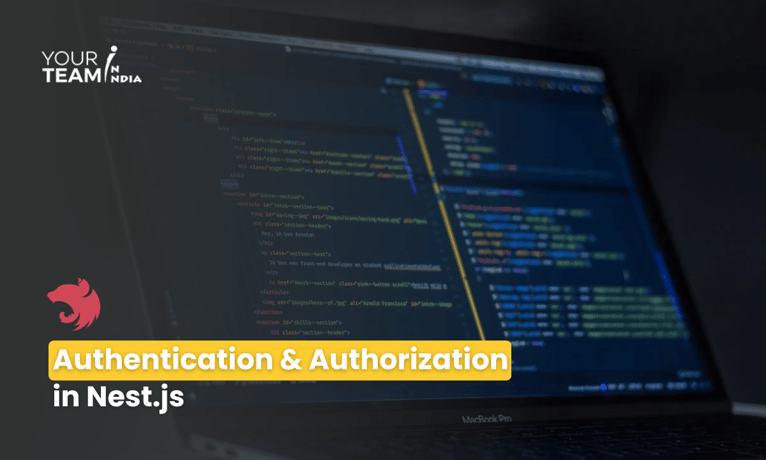
Quick Summary: Delve into the essentials of authentication and authorization in Nest.js. This guide covers fundamental concepts, practical implementation strategies, and best practices to secure your Nest.js applications effectively.
Building secure web apps, especially those created with NestJS—a Node.js framework for creating effective, dependable, and scalable server-side applications—requires careful consideration of authentication and permission. I'll go over the fundamentals of authorization and authentication, how NestJS handles them, and the range of authentication techniques it supports. You'll also get a peek at some code to help you grasp the ideas better.
Introduction to Authorization and Authentication
Authentication is the process of confirming a user's identity and making sure they are who they say they are. What can be done within the application by the authenticated user is determined by authorization after authentication.
Authenticating a NestJS application usually entails confirming the legitimacy of a client-provided token (like a JWT) or validating user credentials (such as a username and password).
On the other hand, authorization entails creating access control rules to limit or allow access to particular resources or features in accordance with the role or permissions of the authenticated user.
Transform Your Vision into Reality with Expert NestJS Developers!
Unlock the full potential of your web applications by hiring our seasoned NestJS developers.
How it Works in Nest.js
Using decorators, guards, and middleware, NestJS offers strong support for building authentication and authorization. Guards safeguard routes by verifying that users have the necessary authorizations before granting access, while middleware intercepts incoming requests to carry out functions like authentication.
Example of Middleware for Authentication:
Example of Guard for Authorization:
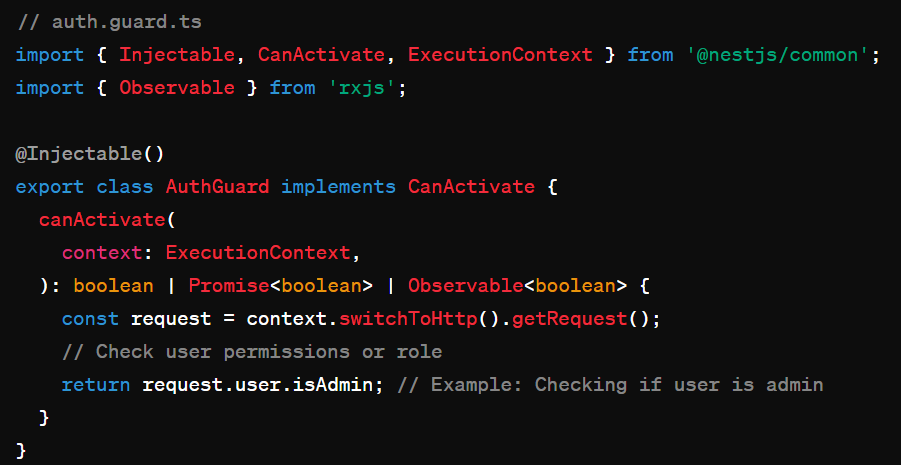
Types of Authentication in NestJS:
There are a number of authentication techniques that NestJS supports, such as:
- JSON Web Tokens (JWT): JWT authentication makes use of JWTs.
Step 1: Install Required Packages
Step 2: Implement JWT Strategy
Step 3: Use JwtAuthGuard for Routes
- Passport.js Integration: Joining Passport with NestJS.JavaScript for methods of authentication like as OAuth, Local, and so forth.
Step 1: Install Required Packages
Step 2: Implement Local Strategy
Step 3: Use LocalAuthGuard for Routes
- OAuth: Setting up third-party authentication providers' OAuth authentication flows.
Step 1: Install Required Packages
Step 2: Configure Google OAuth Strategy
Step 3: Use GoogleAuthGuard for Routes
- Cookies and sessions are used for session-based authentication. NestJS supports session-based authentication out of the box through the '@nestjs/passport' module and the SessionSerializer.
Advantages:
Nest.js's integration of authorization and authentication offers the following benefits:
- Enhanced Security: Nest.js ensures that only authenticated users may access protected resources by implementing both authentication and authorization. Additionally, access is restricted depending on roles or permissions within authenticated users, thus strengthening overall security.
- Granular Access Control: Nest.js gives developers the ability to provide distinct permissions for various application components, providing them with fine-grained control over resource access through authorization mechanisms. Users will only have access to the capabilities they require thanks to this fine-grained management, which also improves security.
- Better User Experience: Authorization makes sure users can access the appropriate features and data according to their role or permissions, while authentication guarantees a smooth login process for users. The customized experience improves customer pleasure and usability.
- Compliance with Regulations: Strict rules governing access control and user data protection are in place in several businesses. Nest.js applications can assure compliance with rules by adding authentication and authorization procedures, hence mitigating legal risks and liabilities.
- Scalability and Maintainability: As the application grows, it will be simpler to design and manage authentication and authorization thanks to Nest.js's built-in support for middleware, guards, and decorators. This integrated support simplifies development, lessens complexity, and makes maintainability easier.
Disadvantages:
Combining permission and authentication in NestJS may have the following drawbacks:
- Complexity: As an application expands, it may become more complex to manage permission and authentication inside the same codebase. Because of its complexity, the codebase may be more difficult to comprehend and update.
- Tight Coupling: The logic governing authorization rules and authentication can become tightly coupled when authentication and authorization are combined. Modifications made to one element could unintentionally impact the other, strengthening the application's fragility.
- Scalability Challenges: Managing authorization and authentication logic simultaneously might get difficult as the programme grows. Performance bottlenecks and scalability problems could result from it, especially if the application has complicated access control requirements or heavy traffic.
- Security Risks: Authentication and authorization together raise the possibility of security flaws. A single weak point (authentication, for example) may jeopardize the authorization system as a whole, exposing confidential information or permitting illegal access.
- Restricted Flexibility: Selecting distinct authentication or authorization solutions separately may be more difficult when authentication and authorization are combined. It can make it more difficult to readily interact with external authentication providers or adjust to changing security requirements.
Conclusion
Finally, middleware, guards, and a variety of authentication techniques are some of the ways that Nest.js offers strong support for authorization and authentication. While permission establishes a user's access rights within an application, authentication entails confirming user identities.
Using techniques like JWT, OAuth, Passport.js integration, and session-based authentication, you can easily integrate authentication and authorization with Nest.js. The overall security and dependability of Nest.js applications are improved by these technologies, which allow for safe user authentication and precise access control.
Developers can adhere to industry best practices for authentication and authorization while creating scalable and secure online apps by integrating these functionalities. All things considered, Nest.js provides an extensive toolkit for building strong authorization and authentication systems, guaranteeing the security and integrity of web applications.
Get reliable, high-performance solutions. Hire Nest.js developers to enhance your team!