Quick Summary: This guide provides an in-depth exploration of essential array methods in React, including map()
, filter()
, reduce()
, and more. It explains how these methods can be effectively used to manipulate and render lists of data in React applications, complete with practical examples and best practices.

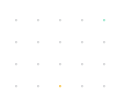
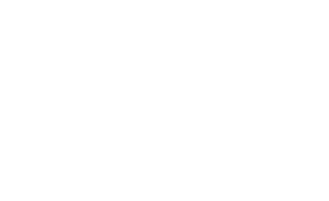
Enhance Your Web Applications with ReactJS
Hire our expert ReactJS developers today to build fast, scalable, and dynamic web applications.
Introduction
In React, managing state often involves dealing with arrays. Array methods in JavaScript become essential tools for manipulating these arrays efficiently. React’s state management encourages immutability, meaning you should avoid directly mutating the state. Instead, you should create and return new arrays when updating the state.
Commonly Used Array Methods
1. map():
The map() method creates a new array populated with the results of calling a provided function on every element in the calling array. This is particularly useful in React for rendering lists of components.
In a React Component
2. filter():
The filter() method creates a new array with all elements that pass the test implemented by the provided function.
In a React Component -
3. reduce()
The reduce() method executes a reducer function (that you provide) on each element of the array, resulting in a single output value.
4. find()
The find() method returns the value of the first element in the provided array that satisfies the provided testing function.
5. findIndex()
The findIndex() method returns the index of the first element in the array that satisfies the provided testing function. Otherwise, it returns -1.
6. some()
The some() method tests whether at least one element in the array passes the test implemented by the provided function.
7. every()
The every() method tests whether all elements in the array pass the test implemented by the provided function.
8. includes()
The includes() method determines whether an array includes a certain value among its entries, returning true or false as appropriate.
9. sort()
The sort() method sorts the elements of an array in place and returns the sorted array. Note that the default sort order is according to string Unicode code points.
10. concat()
The concat() method is used to merge two or more arrays. This method does not change the existing arrays but returns a new array.
Managing State in React with Array Methods
When using these array methods in React, it is crucial to ensure that you do not mutate the state directly. Instead, always create and set new arrays. Here's an example of how to use these methods in a React state management scenario:
Example: Adding an Item to a List
Example: Removing an Item from a List
Example: Updating an Item in a List
Advantages of Array Methods in React
1. Immutability
React's emphasis on immutability aligns well with array methods that return new arrays rather than modifying the existing ones. This leads to several benefits:
- Predictable State Management: By avoiding direct mutations, the state remains predictable and easier to debug.
- Component Re-rendering: React can better detect state changes and optimize component re-rendering, improving performance.
2. Functional Programming Paradigm
Array methods like map(), filter(), and reduce() promote a functional programming style, which has several advantages:
- Readability: Code becomes more concise and easier to read.
- Maintainability: Functional code is often easier to maintain and refactor.
3. Simplified Data Manipulation
Array methods simplify complex data transformations and operations:
- Concise Operations: Methods like map() and filter() allow for concise and expressive operations on arrays.
- Chaining: Methods can be chained together to perform multiple operations in a readable manner.
4. Reusability
Using array methods encourages the creation of reusable functions and components:
- Reusable Components: Components designed to handle arrays can be easily reused across different parts of the application.
- Reusable Logic: Functions that operate on arrays can be abstracted and reused.
5. Enhanced Readability and Expressiveness
Array methods make the code more expressive and easier to understand:
- Declarative Code: Code written using array methods often describes the "what" rather than the "how," making it more declarative.
Disadvantages of Array Methods in React
1. Performance Concerns
While array methods are convenient, they can sometimes introduce performance issues:
- Creating New Arrays: Methods like map() and filter() create new arrays, which can be costly in terms of memory and processing, especially with large datasets.
- Multiple Iterations: Chaining multiple array methods can lead to multiple iterations over the data, which might be inefficient.
2. Learning Curve
There is a learning curve associated with mastering array methods and understanding their implications in React:
- Complexity for Beginners: New developers might find it challenging to understand and use these methods effectively.
- Functional Paradigm: Transitioning from an imperative to a functional programming style can be difficult for those not familiar with it.
3. Immutability Challenges
Enforcing immutability requires careful management of state and arrays:
- Accidental Mutations: Without careful handling, accidental mutations can still occur, leading to unexpected bugs.
- Verbose Code: Ensuring immutability might sometimes result in more verbose code, particularly when performing complex updates.
4. Debugging Difficulties
Debugging issues related to array methods can sometimes be more challenging:
- Chained Methods: When array methods are chained together, it can be harder to pinpoint where an error occurred.
- Asynchronous Issues: Combining array methods with asynchronous operations (e.g., fetching data) can introduce complexity in debugging.
5. Compatibility and Polyfills
Older browsers may not support some modern array methods requiring polyfills:
- Browser Support: Some array methods may not be supported in all environments, necessitating polyfill, which adds extra dependencies.
- Babel and Transpilation: Ensuring compatibility might require using tools like Babel, adding complexity to the build process.
Conclusion
Array methods in React are essential for managing state and rendering dynamic lists efficiently. Key methods like map(), filter(), reduce(), and others allow for effective manipulation of arrays while maintaining immutability.
By creating and returning new arrays instead of mutating existing ones, you ensure better performance and maintainability in your React applications. Understanding and utilizing these methods enables more dynamic and responsive UIs, which is crucial for modern web development.
Need help implementing these Reactjs techniques? Hire our skilled Reactjs developers to assist you in building scalable, fast, and dynamic web applications.